Understanding and Implementing design pattern - FACADE
Introduction
Design patterns are extensively used as a programming methodology to develop extensible and robust applications. In this article, we will discuss and understand a design pattern Facade and evaluate its benefits in making an application scalable.
Background
Let us understand the design pattern with the help of an example.
Consider a Customer ABC who wishes to apply for a house loan from CBC Bank.
public class Customer
{
public string Name { get; set; }
public string Occupation { get; set; }
public Customer(string name, string occupation)
{
Name = name;
Occupation = occupation;
}
}
CBC Bank has a mortgage department which accepts house loan applications and evaluates their eligibility. Note that the customer only contacts the mortgage department to know whether he will be getting the loan or not.
Also, CBC Bank has two subdepartments:
- BankSubsytem that validates the customer background and determines if the customer is a valid customer.
- LoanSubsytem that determines the customer has no bad loans.
public class Bank_Subsystem
{
public bool IsValidCustomer(Customer cust)
{
Console.WriteLine("Check " + cust.Name + " is valid");
return true;
}
}
public class Loan_Subsystem
{
public bool HasNoBadLoan(Customer cust)
{
Console.WriteLine("Check " + cust.Name + " for bad loans");
return true;
}
}
As per CBC Bank policy, the Mortgage department will contact BankSubsystem and LoanSubsystem to determine if the customer is valid and has no bad loans. If the customer is approved by both departments, the Mortgage department reckons he is eligible for a house loan.
public class Mortgage_Facade
{
private Bank_Subsystem bank;
private Loan_Subsystem loan;
public bool IsEligible(Customer cust)
{
bool isEligible = true;
bank = new Bank_Subsystem();
if (!bank.IsValidCustomer(cust))
{
isEligible = false;
}
loan = new Loan_Subsystem();
if (isEligible && !loan.HasNoBadLoan(cust))
{
isEligible = false;
}
return isEligible;
}
}
Note the interesting bit, the current interaction looks like this. The customer is not aware of either of the subsystems and the subsystems are not aware of the customer. Facade (the quintessential middlemen has all the right connections and does the job) knows about both the customer and the subsystems and does the task.
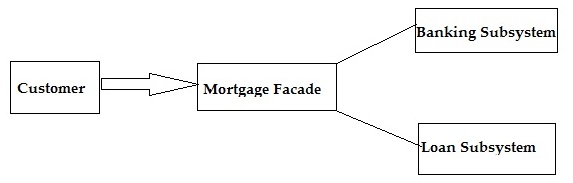
Consider the twist in the tale, a new regulation requires CBC Bank to also check the credit rating of the customer before approving the house loan. So, the Mortgage department should now also contact the credit subsystem which checks the credit worthiness of the customer.
public class Credit_Subsystem
{
public bool HasGoodCredit(Customer customer)
{
Console.WriteLine("Check "+customer.Name + " for good credit");
return true;
}
}
Wow, so what do we do now? Rewrite the entire application from scratch as the bank's policy has changed, or worry about changing each class? Well, absolutely no. Here's the beauty of Facade. We just add the
CreditSubsytem
class to our project and extend Mortgage_Facade.cs.public class Mortgage_Facade
{
private Bank_Subsystem bank;
private Loan_Subsystem loan;
private Credit_Subsystem credit;
public bool IsEligible(Customer cust)
{
bool isEligible = true;
bank = new Bank_Subsystem();
if (!bank.IsValidCustomer(cust))
{
isEligible = false;
}
loan = new Loan_Subsystem();
if (isEligible && !loan.HasNoBadLoan(cust))
{
isEligible = false;
}
credit = new Credit_Subsystem();
if (isEligible && !credit.HasGoodCredit(cust))
{
isEligible = false;
}
return isEligible;
}
}
Note how the relationship between the entities has evolved. The customer still does not know about the subsystems and vice versa. It's the Facade that continues to do the magic.
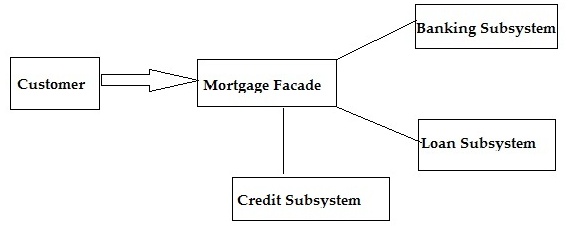